4 Pillars of DSA
4 Pillars of DSA
***********************************************************************************************
1) Procedural Programming Language
2) OOPs (C++,Java,Python etc)
3) STL/JavaCollection/Python DataTypes (list, set,dict, etc)
4) Recursion
***********************************************************************************************
Detailed explanations will be posted on the YouTube channel Soon.
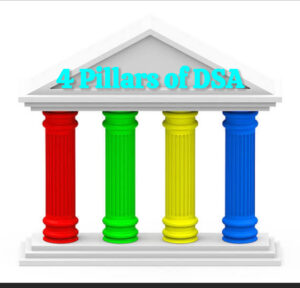
1) Procedural Oriented Programming Language
Overview of Procedural Programming Languages, Advantages & Examples
What Is Procedural Programming?
Procedural programming is a structured programming paradigm based on the concept of procedures
( functions or routines). It is a top-down approach in which applications are split into smaller procedures that are reusable and promote modularization.
Main Characteristics of Procedural Programming
✅ Uses Functions / Procedures – The code is split up into reusable blocks (functions).
✅ Uses a Top-Down Approach – Execution is carried out logically, and step-by-step.
✅ Global and Local Variables – Functions may be aware of local or global data.
Popular Procedural Programming Languages
Language | Key Features | Used In |
---|---|---|
C | Fast, low-level, used in OS, embedded systems | Linux, Windows, IoT |
Pascal | Structured, educational use | Legacy systems, teaching |
Fortran | Scientific computing, high-performance | NASA, weather modeling |
COBOL | Business and banking applications | Banking, legacy finance |
Ada | Safety-critical systems | Aerospace, defense |
BASIC | Beginner-friendly, early computing | Legacy applications |
Go (Golang) | Procedural with concurrency features | Cloud, web services |
Benefits of Procedural Programming
Simplicity – Easy to understand and debug.
Modular Code – Functions improve reusability and maintainability.
Efficient Execution – Faster compared to object-oriented programming in some cases.
Better Control Over Hardware – Useful for system programming.
Easy to Translate into Machine Code – Optimized for performance.
Procedural vs. Other Paradigms
Paradigm | Key Difference |
---|---|
Procedural | Uses functions/procedures to structure code. |
Object-Oriented (OOP) | Uses objects and classes (e.g., Java, C++). |
Functional | Emphasizes immutability and pure functions (e.g., Haskell, Lisp). |
Companies That Use Procedural Programming
4 Pillars of DSA
🏢 Microsoft – Windows kernel and Windows system utilities are daC: [9]
🏢 Apple – macOS and core iOS components in C.
🏢 NASA — Fortran for scientific computing and simulations
🏢 IBM – Cobol – for banking and financial systems
🏢 Google – Uses Golang (Go) extensively for cloud and backend services
Conclusion
Procedural programming is still widely used, especially in system programming, scientific computing, and legacy applications. While newer paradigms like OOP and Functional Programming are popular, procedural languages continue to power critical software worldwide.
2) OOPs (C++,Java,Python etc)
What is OOP?
OOP stands for Object-Oriented Programming, and it is a programming paradigm based on the concept of objects, which may contain data in the form of fields i.e. attributes or properties, and code, in the form of procedures, i.e. methods. They can be thought of as objects that have attributes (data) and methods (behavior), representing real-world entities.
Key Benefits of OOP :
✅ Encapsulated – Packages data and the functions that operate on it, protecting it.
✅ Abstraction – Hides complex implementation details by providing only necessary things.
✅ Inheritance – Reusing the code by creating new classes that inherit some of the properties of existing classes.
✅ Polymorphism -> allows us the flexibility to one function can be used with multiple types.
✅ Modularity & Maintainability — Reusable parts are easier to debug and collaborate on.
✅ Scalability – For large-scale applications like enterprise systems & web applications.
Companies Using OOP
4 Pillars of DSA
🏢 Google – Uses Java, Python, and C++ for scalable applications.
🏢 Microsoft – C# and C++ for Windows, .NET applications.
🏢 Apple – Objective-C and Swift for macOS and iOS development.
🏢 Amazon – Java and C++ for AWS, backend services.
🏢 Facebook (Meta) – PHP, C++, and Hack for web applications.
🏢 Tesla – C++ for autonomous vehicle software.
🏢 Uber – Java and Python for backend services.
OOP: The Backbone of Modern Software Development
Everything You’d Ever Want to Know about (the One and Only) Object-Oriented Programming!
Why OOP is the Future?
OOP dominance is very much visible, as it drives everything from mobile applications to large enterprise systems. The methodologies of object-oriented programming (OOP), which are encapsulation, inheritance, and polymorphism, allow developers to create scalable, reusable, and maintainable codebases.
OOP is a programming paradigm that helps in organizing data effectively when the code base is managed to build a complex web application, an AI-powered system, or a real-time analytics platform, thus allowing for the well-coordinated collection of data by a team of programmers.
3) STL/JavaCollection/Python DataTypes (list, set,dict, etc)
STL (C++), Java Collections, and Python Data Structures: A Comparison
Data structures represent the core of programming, and every programming language offers built-in libraries to handle the data structures efficiently. Here is how they look similar to STL in C++, Collections in Java, and Python data types.
C++ Standard Template Library (STL)
STL provides generic data structures and algorithms.
STL Container | Type | Description |
---|---|---|
vector | Dynamic Array | Resizable array, fast random access. |
list | Doubly Linked List | Fast insertions and deletions. |
deque | Double-Ended Queue | Fast insertions/removals at both ends. |
set | Ordered Set | Unique elements, sorted order. |
unordered_set | Hash Set | Unique elements, no order, fast lookup. |
map | Ordered Dictionary | Key-value pairs, sorted by key. |
unordered_map | Hash Map | Key-value pairs, fast lookup. |
stack | Stack (LIFO) | Last In First Out (LIFO). |
queue | Queue (FIFO) | First In First Out (FIFO). |
priority_queue | Min/Max Heap | Sorted queue (min-heap or max-heap). |
Java Collections Framework
Java provides an extensive Collections Framework for managing data.
Java Collection | Type | Description |
---|---|---|
ArrayList | Dynamic Array | Resizable, fast access. |
LinkedList | Doubly Linked List | Fast insertions/removals. |
HashSet | Hash Set | Unique elements, no order. |
TreeSet | Ordered Set | Unique elements, sorted order. |
HashMap | Hash Table | Key-value pairs, unordered. |
TreeMap | Sorted Map | Key-value pairs, sorted by key. |
Stack | LIFO Stack | Stack implementation. |
Queue | FIFO Queue | Implements Queue interface. |
PriorityQueue | Min/Max Heap | Ordered queue (natural sorting). |
Python Data Structures
Python provides built-in data types that are easy to use.
Python Data Type | Type | Description |
---|---|---|
list | Dynamic Array | Ordered, mutable, allows duplicates. |
tuple | Immutable List | Ordered, immutable, allows duplicates. |
set | Unordered Set | Unique elements, no duplicates. |
dict | Hash Map | Key-value pairs, unordered (ordered in Python 3.7+). |
deque | Double-Ended Queue | Fast insertions/removals at both ends. |
Comparison of STL, Java, and Python
Feature | C++ STL | Java Collections | Python |
---|---|---|---|
Performance | Fastest (low-level) | Slower than C++ (JVM overhead) | Slower than Java (dynamic typing) |
Ease of Use | Medium (complex syntax) | Medium (boilerplate code) | Easiest (built-in, simple syntax) |
Memory Usage | Efficient (manual control) | Higher (JVM overhead) | Higher (dynamic typing, garbage collection) |
Sorting | sort() (O(n log n)) |
Collections.sort() |
sorted() |
Thread Safety | No built-in support | synchronized versions available |
GIL (Global Interpreter Lock) restricts concurrency |
Which One to Use?
4 Pillars of DSA
1) C++ STL is used for high-performance applications, game development, and system programming.
2) For enterprise applications, Android development, and large-scale systems, use Java Collections.
3) Become proficient in Data Structures of Python for Data science, web development, and prototyping.
4) Recursion
Recursion: Use Cases and Applications
What is Recursion?
Recursion is a method of programming in which we create a function that calls itself and better solves the task at hand by dividing the problem into subproblems. It works on the technique of Divide and Conquer.
Key Components of Recursion:
You can learn everything about recursion in just two steps:
✅ Base Case — The condition under the recursion stops
⏸️ Recursive Case — The part of the function to where it calls itself (Modified arguments in the same function call itself)
— Repeat steps 1 & 2 🙌 Recursion has solved our problem.
Use Cases of Recursion
🔹 Mathematical Computations
Factorial (n! = n * (n-1)!)
Fibonacci Series (F(n)=F(n−1)+F(n−2))
🔹 DS & ALGORITHMS
Binary Tree, N-ary TreeTraversals (Tree Traversal)
Graph traversal(DFS — Depth First Search)
Operations on Linked List (Reverse, Search)
Sort Algorithms (Merge Sort, Quick Sort)
🔹 Problem Solving
Backtracking (N-Queens, Sudoku solver, Maze solving)
Dynamic Programming (Recursion with Memoization мотивировано)
Combinatorics (Generating Permutations, Subsets)
🔹 System and OS Level
Recursion in Compilers (Parsing Expression, AST)
Recursive Directory Traversal(Finding files, Searching in Directories)
🔹 AI & Game Development
Minimax Algorithm (AI Decision Making, Chess, Tic-Tac-Toe)
Fractals (Mandelbrot, Koch Snowflake)
Applications of Recursion in Real World
4 Pillars of DSA
🏢 Google – Search Algorithms and AI recursion
🏢 Amazon — for recommendation systems, for sorting vast amounts of data
🏢 Facebook (Meta) — Social Network Analysis with Recursive Graph Algorithms.
🏢 Microsoft — Navigation in compiler name resolutions (static binding) in a whole file system.
🏢 NASA – Recursion on simulation, and astrophysics calculations.
🏢 Game Development — Pathfinding algorithms (A* search, Minimax)
3. Iteration vs. Recursion: When to Use?
Feature | Recursion | Iteration |
---|---|---|
Code Simplicity | More concise, elegant | Often longer, explicit |
Performance | Can be slow (stack overhead) | Usually faster |
Memory Usage | Uses stack memory (risk of stack overflow) | Uses loop variables (more efficient) |
Best Used For | Trees, Graphs, Backtracking | Simple loops, array traversal |
Conclusion
Recursion is a powerful tool in problem-solving, especially for tree-based structures, graph traversals, and backtracking algorithms. While it provides an elegant solution, it must be used carefully to avoid stack overflow and optimize performance.
For More updates on DSA, C++,Interview Questions
Follow us on :
www.youtube.com/@TrendyVSVlogs
www.youtube.com/@VSCodingAcademy
Pingback: 4 Pillars of System Design - Vs Coding Academy